- Joined
- Nov 19, 2014
- Messages
- 2,633
Calibration:
1. Upload the following code to Arduino from IDE.
2. Open Serial Monitor on Arduino IDE. Numbers start to flow. They should be close to zero at this point.
3. Put a known weight on the scale. If it is off from the actual weight, increase or decrease the calibration factor in the line 12.
4. Remove the weight from the scale.
5. Upload the code with a new calibration factor.
6. Repeat 2~5 until the result matches the actual weight.
1. Upload the following code to Arduino from IDE.
Code:
1. /* Calibration sketch for HX711 */
2. #include "HX711.h" // Library needed to communicate with HX711 https://github.com/bogde/HX711
3.
4. #define DOUT 2 // Arduino pin 2 connect to HX711 DOUT
5. #define CLK 3 // Arduino pin 3 connect to HX711 CLK
6.
7. HX711 scale; // Init of library
8.
9. void setup() {
10. Serial.begin(57600);
11. scale.begin(DOUT,CLK);
12. scale.set_scale(1252); // Start scale with a calibration factor. Adjust the factor.
13. scale.tare(); // Reset scale to zero
14. }
15.
16. void loop() {
17. float current_weight=scale.get_units(10); // get average of 10 scale readings
18. Serial.println(current_weight); // Print the result
19. delay(1);
20. }
2. Open Serial Monitor on Arduino IDE. Numbers start to flow. They should be close to zero at this point.
3. Put a known weight on the scale. If it is off from the actual weight, increase or decrease the calibration factor in the line 12.
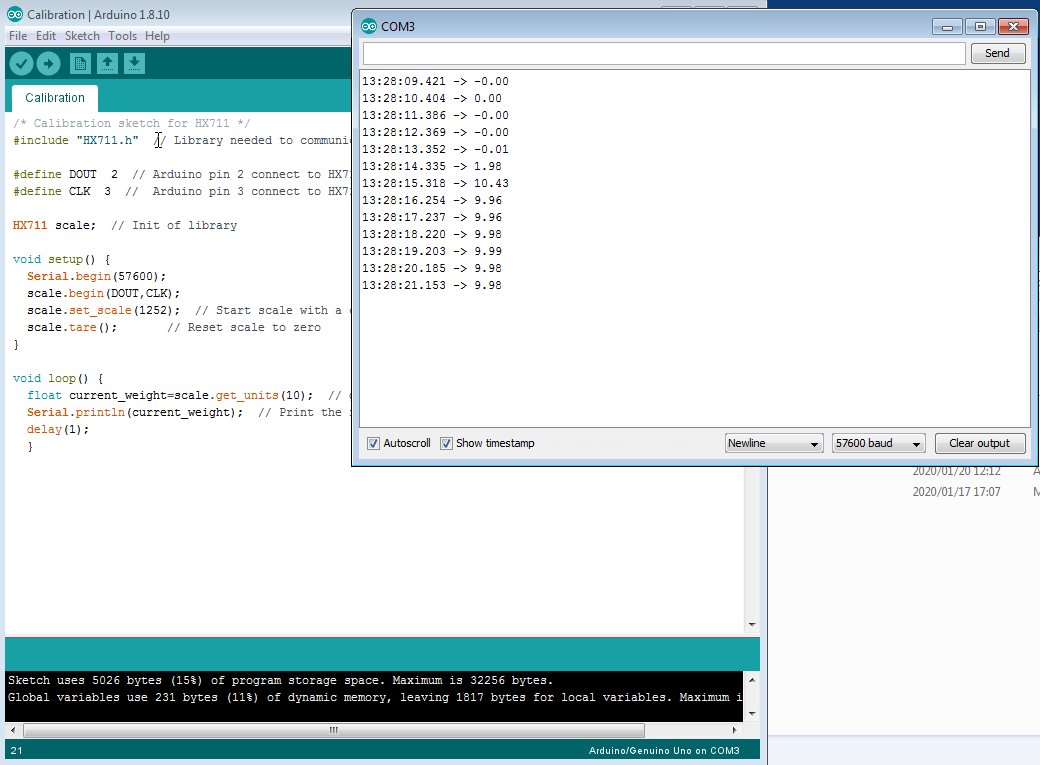
4. Remove the weight from the scale.
5. Upload the code with a new calibration factor.
6. Repeat 2~5 until the result matches the actual weight.